반응형
가운데에 프레임을 띄우는 공식
x = 화면해상도 (scr) - width/2
y = scr - height/2
하지만 setLocationRelativeTo(null); 을 쓰면 자동으로 화면을 맞춰준다
필드 작명법

네이밍 할때는 소문자로 시작해서 _필드이름 으로 작성하여 가독성을 높힐 수 있도록 한다.
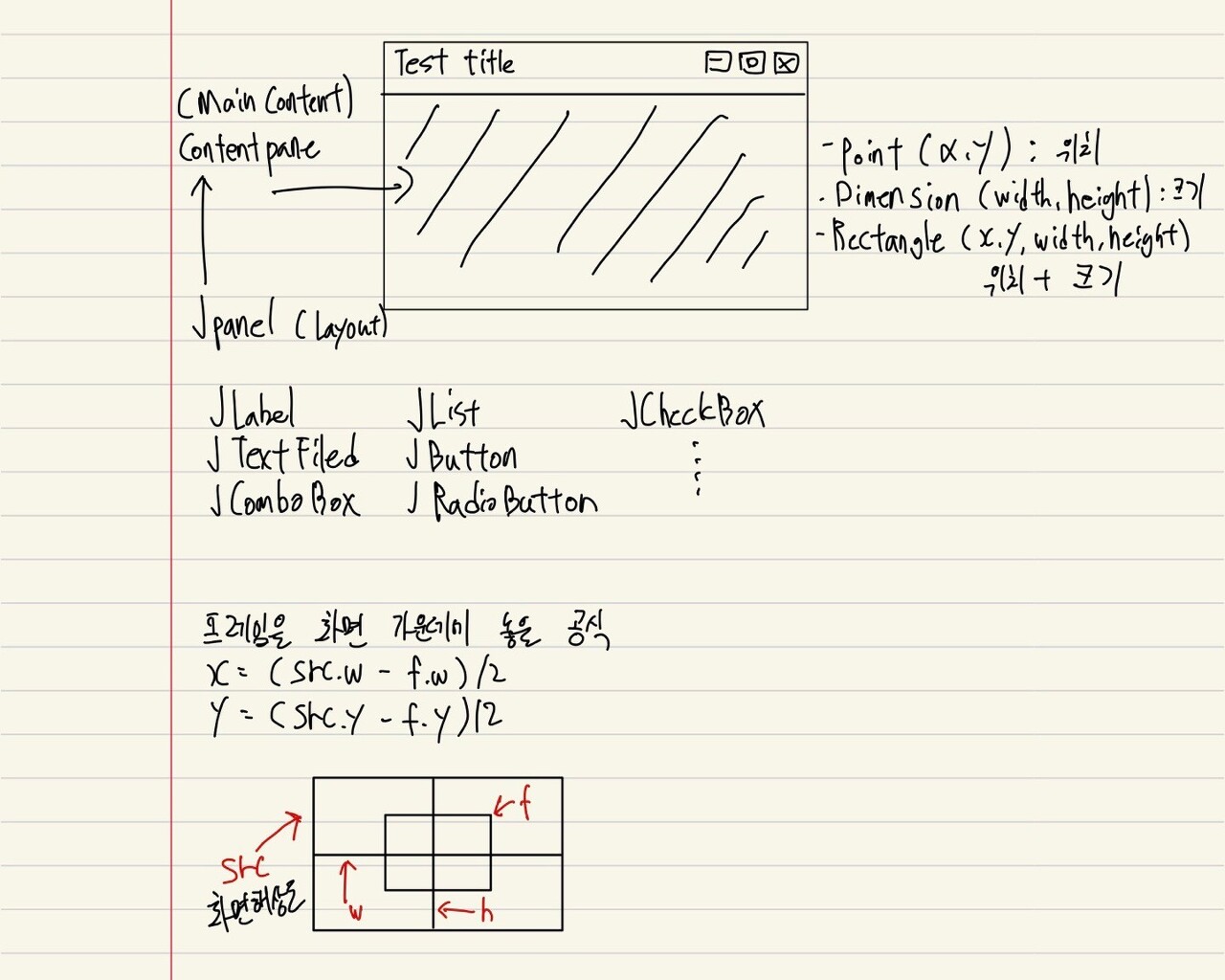
화면구성
Swing 컴포넌트 실습
종류
|
설명
|
BorderLayout
|
JFrame의 기본 레이아웃으로 컴포넌트들을 상,하,좌,우,중앙 으로 배치한다.
|
FlowLayout
|
컴포넌트들을 왼쪽에서 오른쪽으로 일렬로 배치한다. 컨테이너 크기를 넘어서면 자동으로 아래로 배치한다. 생성자로 정렬방식을 지정해줄 수 있다.
|
GridLayout
|
컴포넌트들을 행과 열로 배치한다.
컴포넌트의 크기는 컨테이너의 크기에 자동으로 맞춰진다. |
GridBagLayout
|
컴포넌트들을 행과 열로 배치한다.
각 영역을 서로 다른 크기로 지정해 줄 수 있다. |
BoxLayout
|
행이나 열 방향으로 일렬로 배치한다.
가로 또는 세로로 배치하며 프레임의 끝을 만나도 줄바꿈을 하지 않는다. |
CardLayout
|
한 화면에 여러 컨테이너들을 겹쳐 슬라이드 처럼 사용한다.
하나의 프레임으로 여러 화면을 보여주고 싶을 때 사용한다. |
GroupLayout
|
컨테이너를 순차적 배열과 병렬 배열로 나누고 두개를 결합하여 컴포넌트들을 그룹화한다(?).
|
SpringLayout
|
컴포넌트에 "스프링"을 연결해준다. 컴포넌트들은 다른 컴포넌트를 기준으로 배치된다.
|
BorderLayout
import java.awt.BorderLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
public class BorderLayoutTest extends JFrame {
BorderLayoutTest() {
// BorderLayout은 기본 설정이라 따로 선언하지 않아도 된다.
//setLayout(new BorderLayout());
setSize(500,500);
JButton btns[] = new JButton[5]; // 5개의 버튼 리스트 생성
for (int i = 0; i < btns.length; i++) {
btns[i] = new JButton("버튼" + (i+1));
}
// 상, 하, 좌, 우, 중앙 배치
add(btns[0], BorderLayout.EAST);
add(btns[1], BorderLayout.WEST);
add(btns[2], BorderLayout.SOUTH);
add(btns[3], BorderLayout.NORTH);
add(btns[4], BorderLayout.CENTER);
setDefaultCloseOperation(EXIT_ON_CLOSE); // 창을 닫으면 자동으로 프로세스 종료
setLocationRelativeTo(null); // 창을 가운데에 띄우는 메소드
}
public static void main(String[] args) {
new BorderLayoutTest().setVisible(true);
}
}
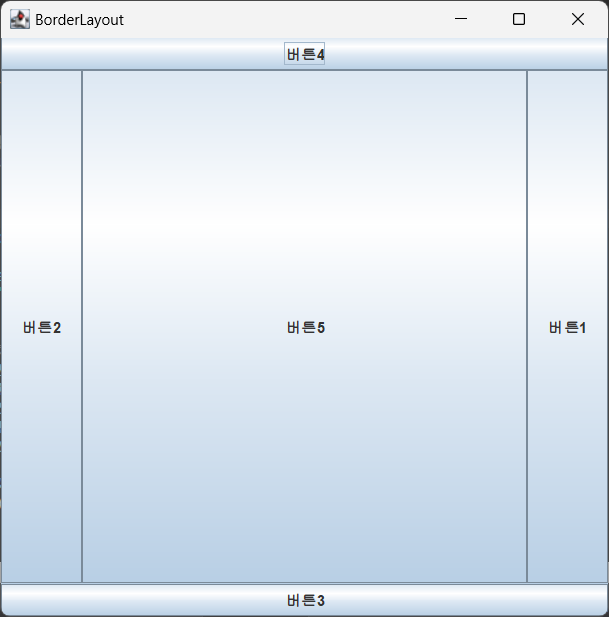
FlowLayout
import java.awt.FlowLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
public class FlowLayoutTest extends JFrame {
FlowLayoutTest() {
setTitle("FlowLayout");
setLayout(new FlowLayout(FlowLayout.CENTER, 15, 15));
// new FlowLayout(int align,int hgap,int vgap) 생성자로 정렬기준 뿐만 아니라 상하좌우 간격도 지정 가능
setSize(500,500);
JButton btns[] = new JButton[5]; // 5개의 버튼 리스트 생성
for (int i = 0; i < btns.length; i++) {
btns[i] = new JButton("버튼" + (i+1));
}
add(btns[0]);
add(btns[1]);
add(btns[2]);
add(btns[3]);
add(btns[4]);
setDefaultCloseOperation(EXIT_ON_CLOSE); // 창을 닫으면 자동으로 프로세스 종료
setLocationRelativeTo(null); // 창을 가운데에 띄우는 메소드
}
public static void main(String[] args) {
new FlowLayoutTest().setVisible(true);
}
}

GridLayout
import java.awt.GridLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
public class GridLayoutTest extends JFrame{
GridLayoutTest() {
setTitle("GridLayout");
setLayout(new GridLayout(3,3)); // 행과 열이 3 X 3
setSize(500,500);
JButton btns[] = new JButton[9]; // 9개의 버튼 리스트 생성
for (int i = 0; i < btns.length; i++) {
btns[i] = new JButton("버튼" + (i+1));
}
add(btns[0]);
add(btns[1]);
add(btns[2]);
add(btns[3]);
add(btns[4]);
add(btns[5]);
add(btns[6]);
add(btns[7]);
add(btns[8]);
setDefaultCloseOperation(EXIT_ON_CLOSE); // 창을 닫으면 자동으로 프로세스 종료
setLocationRelativeTo(null); // 창을 가운데에 띄우는 메소드
}
public static void main(String[] args) {
new GridLayoutTest().setVisible(true);
}
}

주소록 실습
라벨, 텍스트 박스 위치 설정
jPanel_Left.add(jLabel_Name, new GridBagConstraints(0, 0, 1, 1,
1.0, 0.0,
GridBagConstraints.CENTER, GridBagConstraints.BOTH,
new Insets(5, 5, 5, 5),
0, 0));
jPanel_Left.add(jTextField_Name, new GridBagConstraints(1, 0, 1, 1,
1.0, 0.0,
GridBagConstraints.CENTER, GridBagConstraints.BOTH,
new Insets(5, 5, 5, 5),
0, 0));
jPanel_Left.add(jLabel_Name, new GridBagConstraints(0, 0, 1, 1,
1.0, 0.0,
GridBagConstraints.CENTER, GridBagConstraints.BOTH,
new Insets(5, 5, 5, 5),
0, 0));
jPanel_Left.add(jTextField_Name, new GridBagConstraints(1, 0, 1, 1,
1.0, 0.0,
GridBagConstraints.CENTER, GridBagConstraints.BOTH,
new Insets(5, 5, 5, 5),
0, 0));
.
.
. 생략
.
.
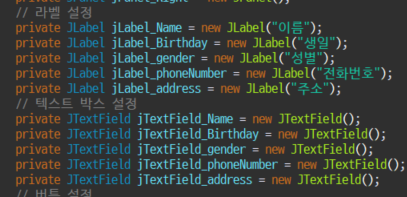
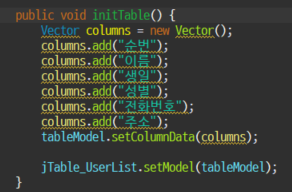
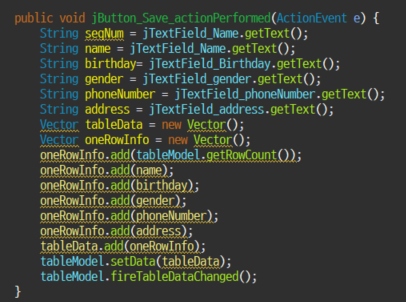
DB와 화면에 출력될 데이터들 값은 일치해야 한다
설정한 데이터 가져오기
private void initData() {
List<AddressBookVo> addressBooklist = new ArrayList<>();
Vector dataList = new Vector<>();
try (SqlSession session = sqlSessionFactory.openSession()) {
AddressBookDao mapper = session.getMapper(AddressBookDao.class);
addressBooklist = mapper.selectAddressBookList(new AddressBookVo());
for (AddressBookVo s : addressBooklist) {
LOGGER.info("onePerson" + s);
Vector data = new Vector();
data.add(s.getSeqNum());
data.add(s.getName());
data.add(s.getBirthday());
data.add(s.getGender());
data.add(s.getPhoneNumber());
data.add(s.getAddress());
dataList.add(data);
}
tableModel.setData(dataList); //값을 불러와서 common 화면에 넣어준다
tableModel.fireTableDataChanged(); // fireTableDataChanged 을 호출하면 테이블 전체에 변경된 데이터가 반영된다
} catch (Exception ex) {
LOGGER.debug(ex.getMessage(), ex);
}
}
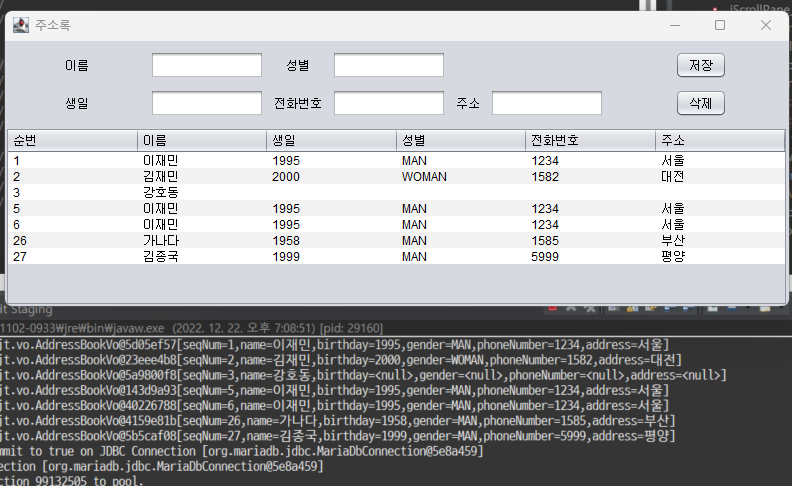
반응형
'인턴' 카테고리의 다른 글
[인턴 OJT 11일차] Swing 아날로그 시계 구현, JTree 주소록 (0) | 2022.12.30 |
---|---|
[인턴 OJT 10일차] 스윙 주소록 저장, 수정, 삭제 / JTree, Thread 정리 (0) | 2022.12.23 |
[인턴 OJT 8일차] Java swing Jframe, Jpanel, Layout (0) | 2022.12.21 |
[인턴 OJT 7일차] mybatis (0) | 2022.12.21 |
[인턴 OJT 6일차] File I/O , DBAddressImpl 수정 및 복습 (0) | 2022.12.20 |